C program to swap two numbers with and without using third variable, swapping in c using pointers, functions (Call by reference) and using bitwise XOR operator, swapping means interchanging. For example if in your c program you have taken two variable a and b where a = 4 and b = 5, then before swapping a = 4, b = 5 after swapping a = 5, b = 4
In our c program to swap numbers we will use a temp variable to swap two numbers.
Swapping of two numbers in c
#include <stdio.h>
int main()
{
int x, y, temp;
printf("Enter the value of x and y\n");
scanf("%d%d", &x, &y);
printf("Before Swapping\nx = %d\ny = %d\n",x,y);
temp = x;
x = y;
y = temp;
printf("After Swapping\nx = %d\ny = %d\n",x,y);
return 0;
}
Download Swap numbers program.
Output of program:
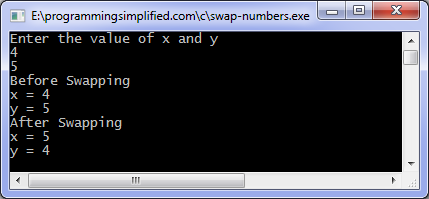
Swapping of two numbers without third variable
You can also swap two numbers without using temp or temporary or third variable. In that case c program will be as shown :-
#include <stdio.h>
int main()
{
int a, b;
printf("Enter two integers to swap\n");
scanf("%d%d", &a, &b);
a = a + b;
b = a - b;
a = a - b;
printf("a = %d\nb = %d\n",a,b);
return 0;
}
To understand above logic simply choose a as 7 and b as 9 and then do what is written in program. You can choose any other combination of numbers as well. Sometimes it's a good way to understand a program.
Swap two numbers using pointers
#include <stdio.h>
int main()
{
int x, y, *a, *b, temp;
printf("Enter the value of x and y\n");
scanf("%d%d", &x, &y);
printf("Before Swapping\nx = %d\ny = %d\n", x, y);
a = &x;
b = &y;
temp = *b;
*b = *a;
*a = temp;
printf("After Swapping\nx = %d\ny = %d\n", x, y);
return 0;
}
Swapping numbers using call by reference
In this method we will make a function to swap numbers.
#include <stdio.h>
void swap(int*, int*);
int main()
{
int x, y;
printf("Enter the value of x and y\n");
scanf("%d%d",&x,&y);
printf("Before Swapping\nx = %d\ny = %d\n", x, y);
swap(&x, &y);
printf("After Swapping\nx = %d\ny = %d\n", x, y);
return 0;
}
void swap(int *a, int *b)
{
int temp;
temp = *b;
*b = *a;
*a = temp;
}
C programming code to swap using bitwise XOR
#include <stdio.h>
int main()
{
int x, y;
scanf("%d%d", &x, &y);
printf("x = %d\ny = %d\n", x, y);
x = x ^ y;
y = x ^ y;
x = x ^ y;
printf("x = %d\ny = %d\n", x, y);
return 0;
}
Swapping is used in sorting algorithms that is when we wish to arrange numbers in a particular order either in ascending order or in descending.